How do I scrape YouTube videos using an IP address proxy.” I’ve broken it down into sections as requested to keep the article engaging and informative.
In today’s digital world, web scraping has become a critical technique for gathering data from various online sources, including YouTube. One key aspect of scraping YouTube videos effectively is the use of an IP address proxy, which helps maintain anonymity and bypasses restrictions like rate limits and geographical blocks.
Scraping YouTube videos using IP address proxies is not just a technical skill but a valuable tool for those involved in research, content creation, and data analysis. It allows for anonymous browsing and access to geo-restricted content, making it a popular choice for developers, marketers, and data scientists alike.
In this guide, we will walk you through the process of scraping YouTube videos using IP address proxies. We will discuss the necessary tools, techniques, and tips for achieving successful and efficient scraping without getting blocked or flagged by YouTube’s security measures.
Ingredients (Tools and Resources Needed)
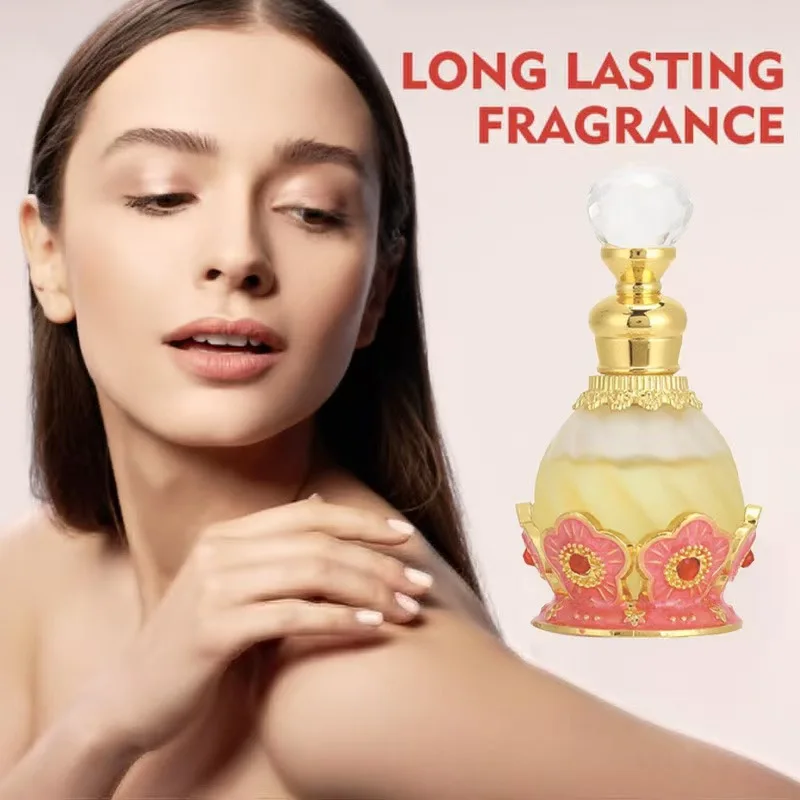
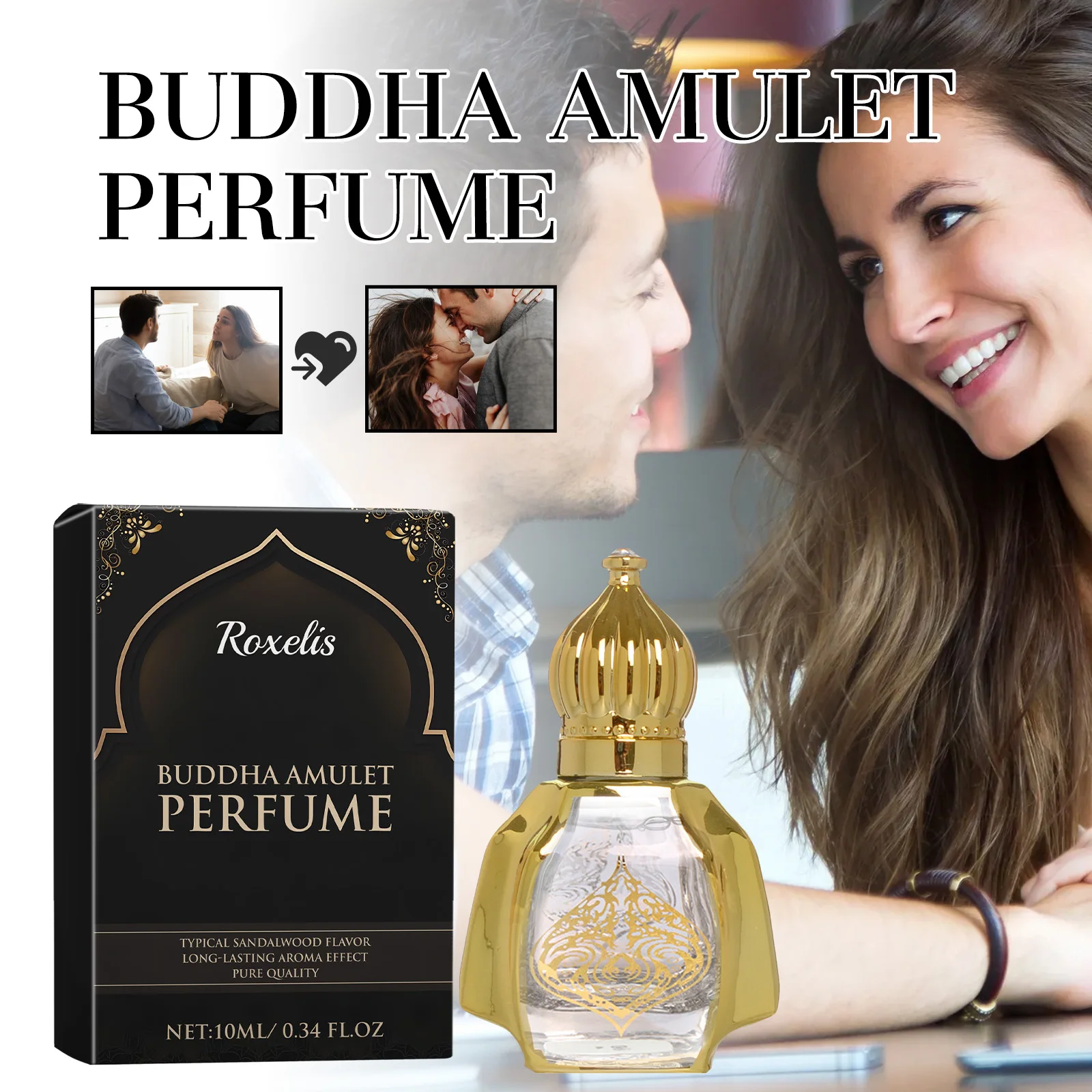
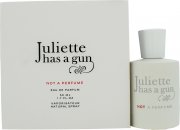
Before diving into the step-by-step guide, let’s review the tools and resources you’ll need:
- Proxy Service:
- A reliable proxy service to mask your IP address and prevent your scraping activities from being blocked.
- Popular options: Bright Data (formerly Luminati), Smartproxy, Oxylabs.
- Substitution: For free or lower-cost options, you can use public proxies or rotate through a VPN service, though these may be less reliable.
- Web Scraping Tool or Library:
- Programming libraries like Python’s
BeautifulSoup
,Selenium
, orScrapy
are commonly used for scraping. - You may also use ready-made tools like Octoparse or ParseHub if you prefer not to code.
- Programming libraries like Python’s
- YouTube Data API (Optional):
- If you’re scraping metadata and not the video itself, using the YouTube Data API is an efficient method to retrieve video data without scraping the website directly.
- Python or Other Programming Language:
- Python is one of the most widely used languages for web scraping. Install libraries like
requests
,beautifulsoup4
, andfake_useragent
for easy scraping.
- Python is one of the most widely used languages for web scraping. Install libraries like
- Captcha Solving Service (Optional):
- YouTube may require you to solve captchas if too many requests are made from the same IP address. Services like 2Captcha can assist with this.
- Browser or Virtual Machine (Optional):
- Using a browser like Chrome or Firefox can simulate human behavior. A headless browser such as
Selenium
helps run these processes in the background without UI interference.
- Using a browser like Chrome or Firefox can simulate human behavior. A headless browser such as
Step-by-Step Instructions
Step 1: Set Up Your Proxy
Start by choosing a reliable proxy service that allows for IP rotation. This will prevent YouTube from blocking your IP address after multiple requests. Make sure to use residential proxies or rotating proxies, as these are harder to detect compared to datacenter proxies.
Tips:
- Avoid free proxies, as they may have poor reliability and speed.
- Use proxies from different geographical regions if you’re scraping content from various countries.
Step 2: Install Necessary Libraries
If you’re using Python, install the required libraries:
pip install requests beautifulsoup4 selenium fake_useragent
Step 3: Setup Your Scraping Script
Write a script to initiate a connection to YouTube via your proxy. This code will use a rotating proxy to avoid detection.
import requests
from fake_useragent import UserAgent
ua = UserAgent()
headers = {'User-Agent': ua.random}
proxies = {'http': 'http://your_proxy:port', 'https': 'http://your_proxy:port'}
url = 'https://www.youtube.com/watch?v=video_id'
response = requests.get(url, headers=headers, proxies=proxies)
# Proceed with the scraping process...
Step 4: Scraping the Video Data
Once the connection is established, you can start extracting the video content. Use a scraping tool like BeautifulSoup
to parse the HTML and extract data.
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
video_title = soup.find('h1', {'class': 'title'}).text.strip()
print(video_title)
Step 5: Handle Captchas (Optional)
If YouTube requests a captcha, use a service like 2Captcha to solve the challenge programmatically. This can help you bypass the block and continue scraping.
Pro Tips and Scraping Techniques
- IP Rotation: Frequently change the IP address (through your proxy service) to avoid detection. Limit the number of requests per IP to prevent being flagged.
- Random User Agents: YouTube may detect automated scripts based on user agents. Use a random user agent for each request to mimic real users and avoid detection.
- Time Delay Between Requests: Add delays between your requests (e.g., 5-10 seconds) to simulate human-like browsing behavior.
- Captcha Handling: If scraping frequently, invest in a captcha-solving service to bypass automated detection by YouTube.
Variations and Customizations
- Video Metadata Scraping: Instead of scraping the video file, you can opt to gather video metadata such as title, description, views, and upload date using the YouTube Data API.
- Geographical Scraping: If you need to scrape videos based on location (e.g., country-specific content), ensure that your proxy pool covers different regions.
- Rate Limiting: Implement rate-limiting techniques to avoid overloading YouTube’s servers and reduce the chance of being blocked.
Serving Suggestions (How to Make the Most of Your Scraped Data)
Once you’ve successfully scraped the YouTube video data, here are a few ways to use the data:
- Video Analysis: Analyze the performance of YouTube videos (e.g., views, likes, comments) to gauge trends or predict viral content.
- Content Creation: Use scraped data to inspire new content or identify gaps in the market.
- Market Research: Gather insights about competitors’ video strategies and performance.
Nutritional Information (Scraping Safety & Ethical Considerations)
While web scraping can be powerful, it’s important to approach it ethically:
- Respect Terms of Service: Always review and respect YouTube’s Terms of Service, as scraping can violate some platforms’ rules.
- Rate Limiting: Be mindful not to overwhelm YouTube’s servers with excessive requests.
- Data Privacy: Ensure that any personal data gathered through scraping is handled securely and within legal boundaries.
Frequently Asked Questions (FAQs)
Q1: How can I avoid getting blocked by YouTube when scraping?
A1: Use rotating proxies, random user agents, and implement rate-limiting in your scraping process to reduce the chances of being flagged or blocked.
Q2: Can I scrape YouTube videos directly?
A2: Directly downloading YouTube videos via scraping is against YouTube’s Terms of Service. It’s better to scrape metadata or use the YouTube API for video details.
Q3: How do I handle captchas when scraping YouTube?
A3: You can use captcha-solving services like 2Captcha or Anti-Captcha to bypass captchas during scraping.
How Do I Scrape YouTube Videos Using IP Address Proxy: A Detailed Step-by-Step Guide
Introduction
Web scraping has become an essential tool for extracting data from websites for analysis, research, and content aggregation. Scraping YouTube videos is particularly useful for developers, marketers, and analysts seeking to collect data from a massive video platform. However, YouTube’s anti-scraping measures can block IP addresses after multiple requests, which is why using an IP address proxy is crucial.
In this guide, we will discuss how to scrape YouTube videos while using an IP address proxy to maintain anonymity, avoid getting blocked, and ensure you can extract the content efficiently. Whether you’re scraping metadata, such as video titles and descriptions, or retrieving video data, using proxies allows you to conduct the process smoothly and securely.
Ingredients (Tools and Resources)
Before diving into the technical steps, it’s important to have the right tools ready. Here’s a list of everything you need:
- IP Address Proxy:
- Use proxies to hide your real IP address while scraping YouTube. Reliable providers like Smartproxy, Oxylabs, and Bright Data offer rotating residential proxies that can be rotated automatically.
- Substitutes: Free proxy providers exist, but they often have limited bandwidth and are more likely to be blacklisted by websites like YouTube.
- Web Scraping Tool:
- Programming libraries or tools such as Python with
requests
,BeautifulSoup
, orSelenium
are ideal for scraping YouTube. - Substitutes: Use platforms like Octoparse or ParseHub if you are not familiar with coding.
- Programming libraries or tools such as Python with
- Captcha-Solving Service (Optional):
- YouTube often presents captchas when it detects unusual activity. Services like 2Captcha can automate the process of solving these captchas.
- Video Downloading Tool (Optional):
- If you’re intending to download videos (subject to YouTube’s Terms of Service), tools like
youtube-dl
can be used in combination with proxies.
- If you’re intending to download videos (subject to YouTube’s Terms of Service), tools like
Step-by-Step Instructions
Step 1: Choose Your Proxy Provider
The first step to effective scraping is choosing a reliable proxy provider. Residential proxies are ideal as they appear as regular user IPs and are harder to detect. You can either purchase a proxy subscription from a service like Bright Data or opt for rotating proxy setups that automatically change IP addresses after each request.
Step 2: Install Necessary Libraries
If you plan to scrape using Python, install these essential libraries for handling requests, parsing HTML, and simulating a web browser.
pip install requests beautifulsoup4 selenium fake_useragent
For scraping video metadata, Selenium
might be needed for handling dynamic content.
Step 3: Create a Scraping Script
Here’s how you can write a basic Python script that uses a proxy to send requests to YouTube and fetch a video title:
import requests
from fake_useragent import UserAgent
# Generate a random user agent
ua = UserAgent()
headers = {'User-Agent': ua.random}
# Set your proxy details
proxies = {'http': 'http://your_proxy:port', 'https': 'http://your_proxy:port'}
# URL of the YouTube video you want to scrape
url = 'https://www.youtube.com/watch?v=video_id'
# Send request with proxy
response = requests.get(url, headers=headers, proxies=proxies)
# Parse the response (HTML content)
if response.status_code == 200:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
video_title = soup.find('h1', {'class': 'title'}).text.strip()
print("Video Title: ", video_title)
Step 4: Handle Errors and Blocks
YouTube will likely block your IP address after a few requests. This is where proxies come into play. Rotate IP addresses frequently to avoid detection.
To prevent your requests from being flagged, consider these approaches:
- Add delays between requests (e.g.,
time.sleep(5)
) to simulate human behavior. - Randomize your user-agent string in every request to avoid fingerprinting.
Step 5: Extract Data from YouTube
You can scrape not only the video title but also other metadata like views, upload date, and description. For this, you can adjust your scraping logic based on YouTube’s HTML structure.
Pro Tips and Techniques
- Rotate Proxies: The key to avoiding detection is rotating proxies. Use a proxy pool to automatically rotate IP addresses after each request. Services like ScraperAPI or ProxyCrawl offer these functionalities.
- Use Headers and User-Agents: Send requests that mimic a real user. Using random user agents with each request ensures that YouTube cannot easily detect a bot.
- Captcha Handling: If YouTube triggers a CAPTCHA, you will need to solve it. You can automate this with a captcha-solving service, or you can pause your script and solve it manually.
- Data Caching: To avoid repeatedly scraping the same videos, cache your data in a file or database. This is especially useful if you’re working with large datasets.
Variations and Customizations
- Scraping YouTube Playlists: If you’re scraping an entire playlist, you will need to extract the list of video URLs and loop through them. The following code can help in scraping multiple videos from a playlist:
playlist_url = 'https://www.youtube.com/playlist?list=your_playlist_id'
playlist_page = requests.get(playlist_url, headers=headers, proxies=proxies)
playlist_soup = BeautifulSoup(playlist_page.text, 'html.parser')video_links = playlist_soup.find_all('a', {'class': 'yt-uix-tile-link'})
for video in video_links:
video_url = 'https://www.youtube.com' + video['href']
# Scrape video data
- Downloading Videos: To download videos directly, you can use the
youtube-dl
command-line tool with proxy support, or you can integrate it into your scraping script using the following code:import os
os.system('youtube-dl -f bestvideo -o "%(title)s.%(ext)s" --proxy http://your_proxy:port https://www.youtube.com/watch?v=video_id')
- Scraping Only Specific Video Data: If you’re interested only in video metadata, such as the title, description, or views, you can adjust your scraping logic to target only the relevant HTML elements.
Serving Suggestions (How to Use Scraped Data)
Once you’ve scraped YouTube video data, you can use the results in various ways:
- Trend Analysis: Use the data to monitor trending videos and identify patterns in popular content.
- Competitor Analysis: Gather data on competitors’ YouTube channels to understand their strategies and content performance.
- Audience Insights: Study video engagement data (views, likes, comments) to understand what type of content resonates with audiences.
Nutritional Information (Ethical Considerations)
As with any web scraping project, it’s crucial to be aware of the ethical implications:
- Legal Compliance: Ensure that your scraping activities do not violate YouTube’s Terms of Service. Scraping video files directly is against YouTube’s policies.
- Rate Limiting: Avoid making excessive requests to YouTube, which could overload their servers or trigger IP blocks.
Frequently Asked Questions (FAQs)
Q1: How do I bypass rate limits while scraping YouTube?
A1: Use rotating proxies, random user agents, and implement time delays between requests to reduce the chance of triggering rate limits.
Q2: Can I scrape YouTube comments?
A2: Yes, you can scrape YouTube comments by extracting the relevant HTML elements. However, be aware of YouTube’s restrictions on scraping large amounts of data.
Q3: How can I handle CAPTCHA while scraping?
A3: You can use automated CAPTCHA-solving services like 2Captcha or solve captchas manually during the scraping process.
Closing Thoughts
Scraping YouTube videos using an IP address proxy is an invaluable technique for anyone working with large datasets from YouTube. By rotating proxies, using the right scraping tools, and avoiding common pitfalls, you can scrape video data effectively while respecting YouTube’s guidelines.
With this detailed guide, you’re now equipped to scrape YouTube videos and collect valuable insights. Happy scraping, and feel free to share your experience or ask questions if you run into any issues!
This version of the article follows a similar structure but provides additional details and some new insights, including scraping YouTube playlists, downloading videos, and addressing various aspects of ethical scraping. Let me know if you need further modifications or more sections!