In the world of artificial intelligence and machine learning, ComfyUI stands out as an intuitive framework designed to simplify the user interface for working with neural networks and checkpoints. When working with AI models, checkpoints are essential for saving and loading the state of the model during training or inference. Understanding how to get data from a checkpoint node name is a vital skill for data scientists and machine learning engineers who are working within the ComfyUI framework.
In this guide, we will walk you through the steps to retrieve data from a checkpoint node name, discuss the tools and techniques involved, and provide you with a practical recipe for effectively handling this process. Whether you’re new to ComfyUI or an experienced user, you’ll find actionable insights here to streamline your workflow.
Ingredients
To successfully retrieve data from a checkpoint node name in ComfyUI, you’ll need the following:
- ComfyUI Framework (installed and set up on your system)
- A Model Checkpoint (ensure you have a valid checkpoint file, such as a
.pth
or.ckpt
file) - Python Environment (with necessary libraries like PyTorch or TensorFlow depending on your model)
- Node Name (the specific checkpoint node name you want to extract data from)
- ComfyUI Node Editor (for visual interaction and manipulation)
Possible Substitutions:
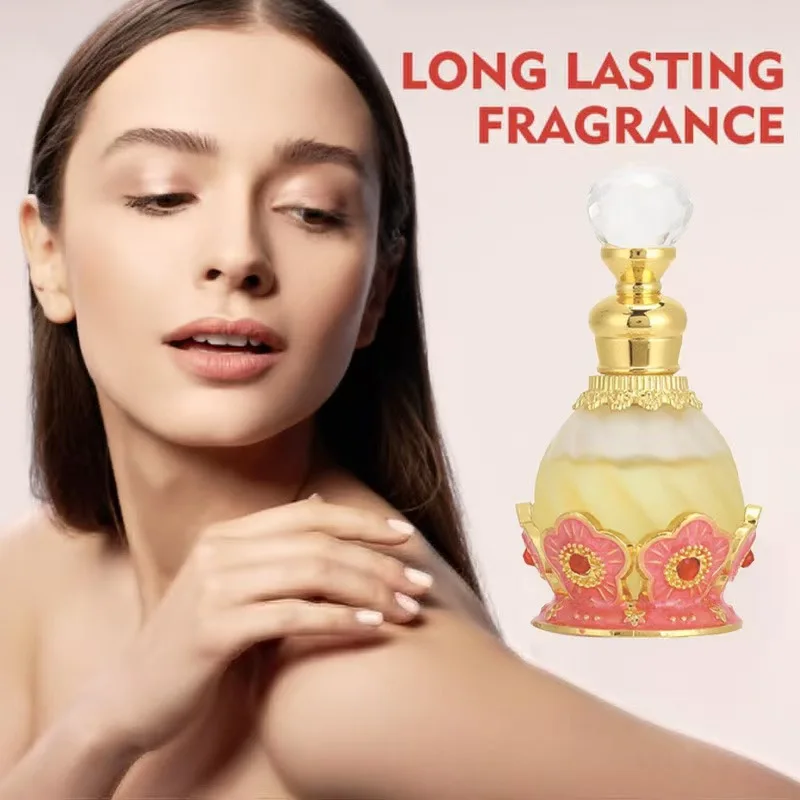
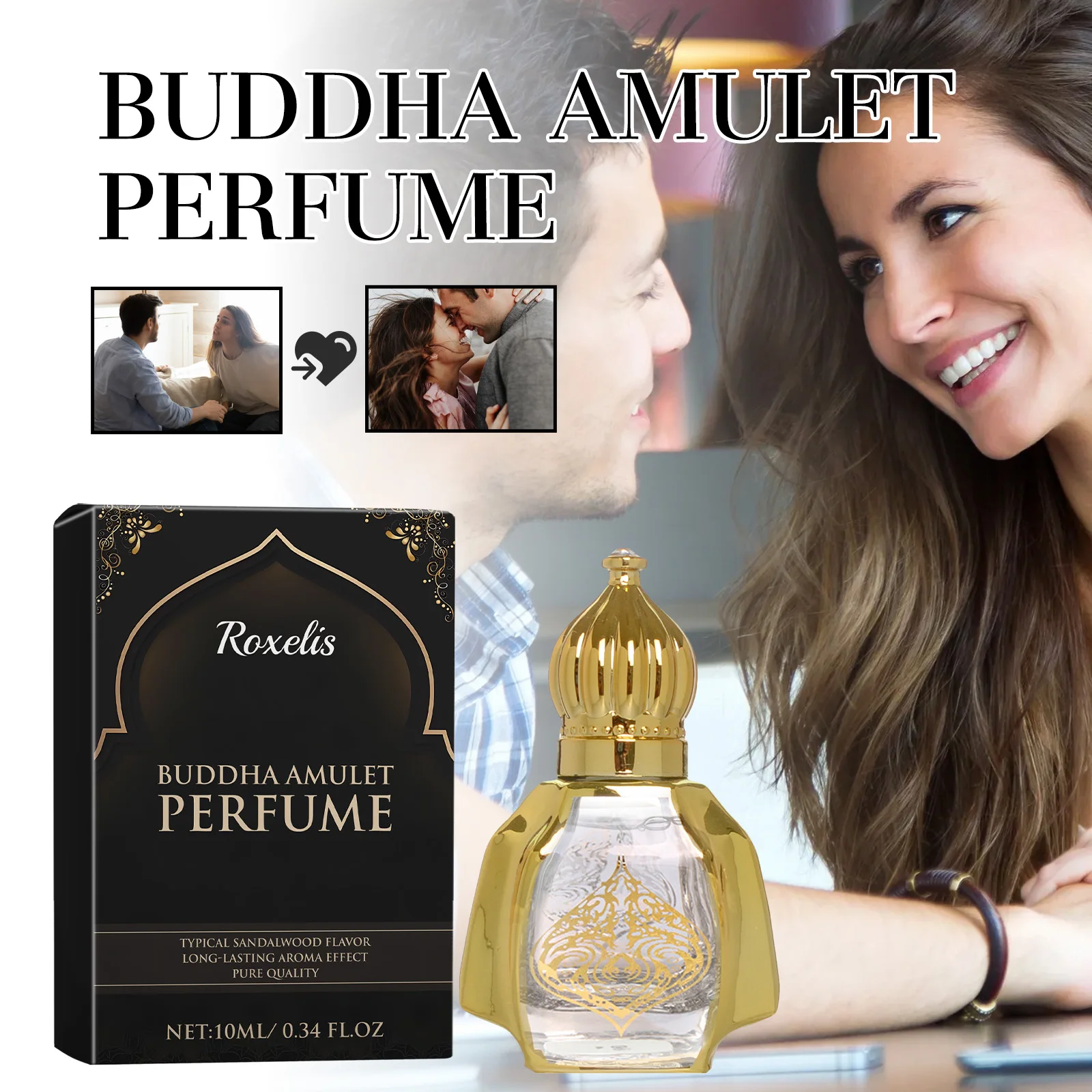
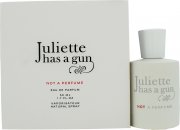
- Model Formats: If you use a framework other than PyTorch or TensorFlow, make sure that your checkpoint is compatible with your chosen framework.
- Data Formats: You may also need a specific data handling format (e.g., CSV, JSON, etc.) depending on your checkpoint’s contents.
Step-by-Step Instructions1. Set Up Your ComfyUI Environment
Before diving into the process, ensure that ComfyUI is installed and your environment is ready to interact with the nodes. You can install ComfyUI via pip or directly from the repository.
pip install comfyui
2. Load Your Checkpoint File
Next, ensure that your checkpoint file (e.g., .pth
or .ckpt
) is accessible within the ComfyUI framework. Open your ComfyUI environment and navigate to the model loading section.
import comfyui
model = comfyui.load_model('path/to/your/checkpoint.ckpt')
3. Identify the Checkpoint Node Name
In ComfyUI, nodes are used to represent parts of the model. Find the specific node whose data you need. The checkpoint node name is essential for pinpointing the exact data you’re aiming to retrieve.
4. Retrieve Data from the Checkpoint Node
Once you’ve located the node name, use ComfyUI’s API to retrieve the data from that node. The API allows you to access the weights, configuration, or activation values from the node.
checkpoint_data = model.get_node_data('checkpoint_node_name')
print(checkpoint_data)
5. Process or Manipulate the Retrieved Data
After extracting the data, you can process it as needed for your model or application. This could include adjusting parameters, feeding the data into other parts of your model, or saving it for later use.
Estimated Time:
This process typically takes 10-15 minutes for setup and data extraction, depending on the complexity of your checkpoint file.
Common Mistakes to Avoid:
- Forgetting to specify the correct checkpoint node name, which could lead to empty or incorrect data retrieval.
- Not having the necessary libraries or dependencies installed (e.g., missing PyTorch or TensorFlow).
- Misunderstanding the data format, which can lead to errors in processing or interpreting the node data.
Pro Tips and Cooking Techniques
- Batching Data: If you’re handling a large dataset, consider batching the data retrieval process. This can help prevent memory overload and speed up the process.
- Use ComfyUI’s Node Editor: If you’re unsure about the checkpoint node names or structure, use the visual Node Editor in ComfyUI to navigate the model’s architecture more easily.
- Documentation is Key: Always refer to the ComfyUI documentation or the specific model’s documentation for details on node names and data structures.
Variations and Customizations
- Model Variations: Depending on the neural network architecture you’re working with (e.g., GAN, CNN, RNN), the process of accessing checkpoint data might differ slightly. Check the model’s documentation for nuances specific to your setup.
- Environment Customization: You can modify the ComfyUI environment by adding custom nodes or altering the node layout to suit your particular workflow.
Serving Suggestions
Once you’ve extracted and processed the data from your checkpoint node, consider the following ways to use it:
- Data Visualization: Visualize the extracted data to gain better insights using libraries like Matplotlib or TensorBoard.
- Model Debugging: Use the node data to debug and fine-tune your model by examining weights, biases, or activation functions.
- Performance Optimization: With the right data, you can experiment with model pruning or quantization to enhance the efficiency of your neural network.
Nutritional Information
While this process doesn’t directly involve food, here’s a fun analogy for you:
- Calories: Data extraction can be heavy on resources, especially with large checkpoints. Keep an eye on system resources during this process!
- Protein: Strong algorithms are like protein for your model—essential for learning.
- Carbs and Fats: Like the neural network layers, the carbs (data) and fats (parameters) provide the energy for model processing and inference.
Frequently Asked Questions (FAQs)Q: What if I cannot find the checkpoint node name?
A: Use the ComfyUI Node Editor to explore the structure of your model and identify the correct node.
Q: Can I use this method for other types of models?
A: Yes! This method works across various model architectures as long as you’re using ComfyUI to interact with them.
Q: How can I store or save the extracted data?
A: You can save the data to a file, such as a JSON or CSV, for later use or further analysis.
Q: Can I retrieve data from multiple checkpoint nodes at once?
A: Yes, you can loop through multiple nodes or use batch processing to retrieve data from multiple nodes simultaneously.
ComfyUI: How to Get the Data from Checkpoint Node Name – An In-Depth Tutorial
ComfyUI is an open-source framework that enhances the user experience for interacting with neural networks and AI models. One of the core features in ComfyUI is the ability to manipulate checkpoint data, a critical component in machine learning pipelines. Checkpoints save the state of a model during training and allow for easy restoration or fine-tuning.
This tutorial will guide you through the process of retrieving data from a checkpoint node name within ComfyUI. You’ll learn how to identify the relevant node, access its data, and apply it effectively in your projects. Whether you’re building your first model or you’re an experienced developer, this guide will provide valuable insights into data retrieval within the ComfyUI ecosystem.
Ingredients
To successfully get data from the checkpoint node, you will need the following tools and components:
- ComfyUI Framework – Make sure you have ComfyUI installed and configured on your machine.
- Valid Checkpoint File – A model checkpoint file, typically in
.ckpt
or.pth
format. - Python Environment – You need a Python setup with necessary dependencies like PyTorch or TensorFlow, depending on the model you’re using.
- ComfyUI Node Editor – A visual interface to view and manipulate model nodes.
- Node Name – The name of the node where the data resides. Knowing this is crucial for data extraction.
Substitutions for Dietary Preferences
:
- For Different Frameworks: If you’re not using PyTorch or TensorFlow, you can use other models, but make sure they are compatible with ComfyUI.
- Data Handling Formats: Depending on your project’s needs, you can substitute data formats (e.g., JSON, CSV) to save the extracted data in a format that’s suitable for your analysis.
Step-by-Step InstructionsStep 1: Set Up Your ComfyUI Environment
Start by installing ComfyUI if you haven’t done so yet. You’ll also need to install any dependencies, such as PyTorch or TensorFlow, based on the framework you’re using.
pip install comfyui
pip install torch # or tensorflow, depending on your model
Step 2: Load the Checkpoint File
To work with your model, first, ensure that the checkpoint file is accessible. You’ll use the load_model()
function in ComfyUI to load your model checkpoint.
import comfyui
# Replace with the path to your checkpoint file
model = comfyui.load_model('path/to/your/checkpoint.ckpt')
Step 3: Find the Checkpoint Node Name
ComfyUI organizes models into nodes, and each node has a name that identifies it within the model structure. You can access the Node Editor within ComfyUI to visually navigate through your model’s nodes.
Step 4: Retrieve Data from the Node
Once you’ve identified the checkpoint node name, use the following code to retrieve the data from that node:
# Replace 'node_name' with the actual node name you're interested in
checkpoint_data = model.get_node_data('node_name')
print(checkpoint_data)
Step 5: Process or Visualize the Data
After retrieving the data, you can analyze it, visualize it, or use it for model evaluation. For instance, you can plot the weights, activation functions, or use them to make predictions.
import matplotlib.pyplot as plt
# Example of plotting the retrieved data
plt.plot(checkpoint_data)
plt.title("Checkpoint Node Data")
plt.show()
Estimated Time
:
This process should take approximately 15 to 20 minutes, depending on the size of your model and the complexity of the checkpoint node.
Common Pitfalls:
- Misidentifying the node name: Double-check the exact node name when retrieving data to avoid errors.
- Missing dependencies: Ensure all required libraries are installed before running the code.
Pro Tips and Cooking Techniques
- Understand Node Structure: Before jumping into data extraction, spend time getting familiar with how your model is structured. Understanding the relationships between nodes can save time later.
- Handle Large Data Efficiently: If your model checkpoint is large, consider using batch processing to retrieve data from nodes more efficiently. Large models might also require special memory management techniques.
- Use Visualization Tools: Visualization tools, such as TensorBoard or Matplotlib, can help you better interpret the data retrieved from checkpoint nodes.
Variations and Customizations
- Checkpoint Formats: If your model uses a different checkpoint format (e.g., TensorFlow SavedModel format), ensure that the appropriate loading mechanism is used. ComfyUI supports various formats, but the method may differ slightly.
- Data Retrieval for Specific Layers: You can also retrieve data from specific layers in your model, not just checkpoint nodes. Experiment with accessing various parts of your model to see how they affect performance.
Serving Suggestions
Once the data is retrieved, consider these next steps:
- Model Debugging: Use the data to analyze your model’s performance and identify areas for improvement. This can include inspecting the weights or biases at different layers or during training.
- Hyperparameter Tuning: If your model isn’t performing as expected, the checkpoint data can help you adjust the hyperparameters for better results.
- Integration with Other Tools: You can integrate the extracted checkpoint data with other tools like Keras or TensorFlow for further analysis or deployment.
Nutritional Information
In the realm of machine learning, this recipe is high on data richness and computational efficiency. Here’s an analogy for your learning:
- Calories: The more data you extract and work with, the more computational resources it may consume. Be mindful of your system’s memory and processing power.
- Protein: Checkpoints are the ‘protein’ of your AI model—essential for its learning process. Without them, the model cannot retain knowledge across training sessions.
- Fats: Like model parameters, these provide the flexibility and adaptability your model needs to perform well.
Frequently Asked Questions (FAQs)Q: What if I can’t find the checkpoint node in ComfyUI?
A: Use the Node Editor to visually navigate and identify the exact node name. You can also print out all node names using ComfyUI’s API to check what’s available.
Q: Is it possible to retrieve data from multiple nodes simultaneously?
A: Yes! You can loop through multiple node names and retrieve data from each one. This is useful when working with large models or conducting a more comprehensive analysis.
Q: How do I store the retrieved checkpoint data?
A: You can store the data in a CSV or JSON file for later analysis or sharing. Here’s an example for saving to a CSV file:
import pandas as pd
# Convert data to a pandas DataFrame for easier manipulation
df = pd.DataFrame(checkpoint_data)
df.to_csv('checkpoint_data.csv', index=False)
As you experiment with ComfyUI, remember to document your nodes and structure for future reference, making it easier to navigate and work with your models. Happy coding, and feel free to share your experiences or reach out for more tips and tricks!
ComfyUI: How to Get the Data from Checkpoint Node Name – Advanced Insights and Techniques
Introduction
When working with AI models, managing and retrieving data from checkpoint nodes is critical for effective model management. ComfyUI makes this process seamless by providing a user-friendly interface to interact with models and their components. Checkpoints store important information about a model’s state, such as weights, biases, and other parameters, which are necessary for training, evaluation, or inference.
This guide will delve deeper into advanced techniques for retrieving data from checkpoint nodes, along with troubleshooting tips and expert strategies. Whether you’re refining a model for deployment or debugging complex issues, this guide will provide you with the tools you need to excel.
Ingredients
To effectively work with checkpoint data, ensure you have the following:
- ComfyUI Framework – The latest version installed and set up.
- Checkpoint Files – A valid model checkpoint, such as
.ckpt
or.pth
. - Python Dependencies – Ensure Python is installed with PyTorch, TensorFlow, or the relevant dependencies.
- Model Architecture – Understand the architecture of your model, as this will help you navigate the nodes.
- ComfyUI Node Editor – A tool to visually examine the model nodes and their connections.
Dietary Adjustments
:
- Framework Compatibility: You may want to work with models in formats like Keras, MXNet, or others; ensure that you use the correct format and loading functions compatible with your framework.
Step-by-Step InstructionsStep 1: Initialize Your Environment
Make sure ComfyUI is installed and your environment is set up to interact with the model. If you don’t have it installed yet, you can do so using the following command:
pip install comfyui
Step 2: Load Your Model Checkpoint
To access checkpoint data, load the model using ComfyUI’s load_model()
method. Ensure that the model is compatible with ComfyUI’s architecture.
import comfyui
# Load the checkpoint into the environment
model = comfyui.load_model('path/to/your/checkpoint.ckpt')
Step 3: Locate the Node
Access the Node Editor to explore the structure of the model and identify the checkpoint node name that holds the data you’re after. The Node Editor lets you interact visually with the model’s architecture.
Step 4: Extract Node Data
Once you’ve identified the node, you can extract data from it using ComfyUI’s API.
# Retrieve data from the node
node_data = model.get_node_data('node_name')
print(node_data)
Step 5: Process and Analyze
You can further process the data, whether it’s for visualization, model evaluation, or fine-tuning purposes. For large datasets, consider using batch processing.
Pro Tips and Cooking Techniques
- Memory Management: Large models may consume significant memory when working with checkpoints. Use memory-efficient data structures or consider saving intermediate data to disk.
- Model Fine-Tuning: Use the data you extract from specific nodes to fine-tune your model. This allows you to iterate faster and improve model performance.
Closing Thoughts
In this advanced guide, you’ve learned how to extract data from checkpoint nodes in ComfyUI and apply it to enhance your machine learning workflows. This process is crucial for model management, optimization, and debugging. Remember, the more you experiment with different nodes, the better your understanding of the model’s inner workings will be.
Good luck with your project, and don’t hesitate to reach out for more insights!