Populating a form with JSON data is a common requirement in modern web development, especially when working with frameworks like DevExtreme. DevExtreme provides a powerful set of UI controls for web applications, and using its MVC form component in combination with JSON data can simplify the process of dynamically populating form fields. This guide explains how to use JSON strings to populate DevExtreme MVC form data, offering step-by-step instructions for both beginners and experienced developers.
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that is easy for both humans and machines to read and write. Integrating JSON with your DevExtreme MVC form enables you to create dynamic, data-driven applications that respond quickly to user input.
Ingredients
-
DevExtreme MVC form control: The main component to be populated.
-
JSON string: The data format that you will use to populate the form.
-
JavaScript: The programming language used to parse the JSON and interact with the DevExtreme form.
-
C# (MVC): The backend code to handle data processing and API requests.
-
jQuery: For simplifying DOM manipulations (optional).
Substitutions
-
If you prefer using Angular or React for frontend development, the general approach remains the same but with different frameworks and tools (e.g., Angular Forms, React State management).
Step-by-Step Instructions
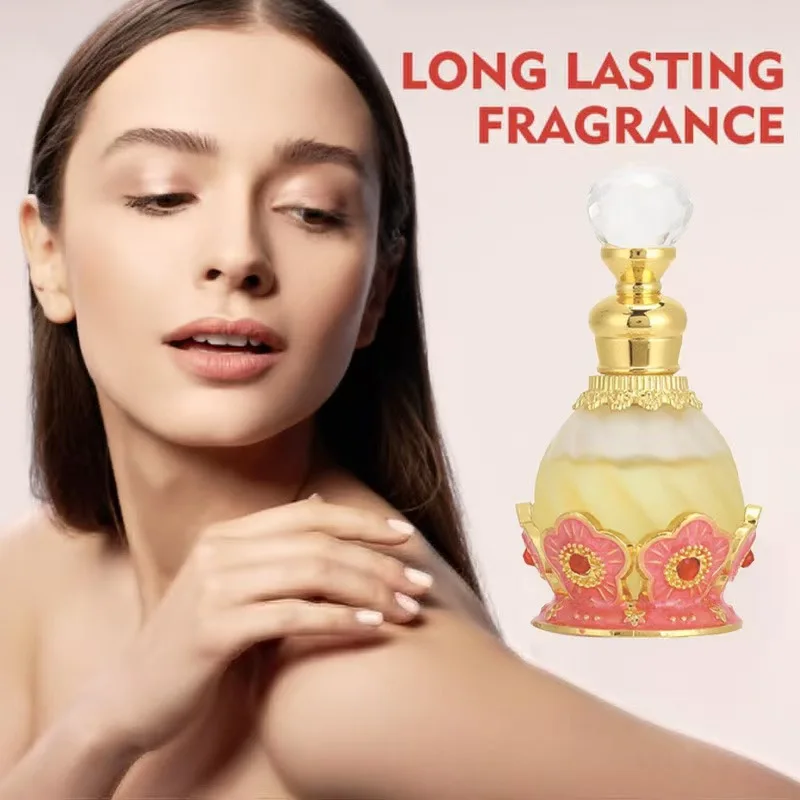
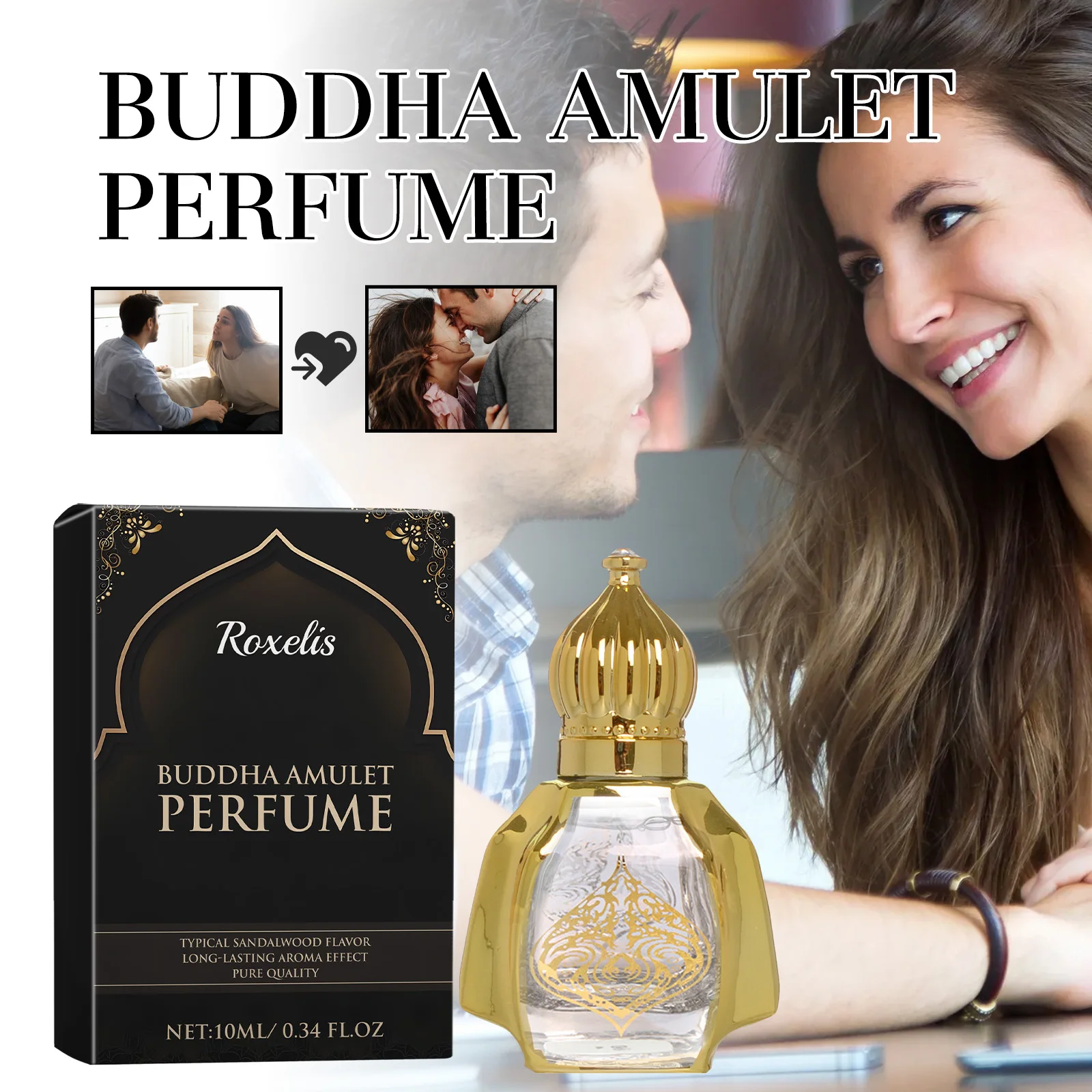
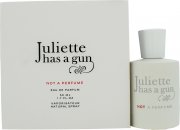
Step 1: Set Up Your MVC Project
-
Create a new MVC project in Visual Studio (or your preferred IDE).
-
Install DevExtreme via NuGet Package Manager or by including it in your project’s scripts.
-
In your Layout.cshtml file, include the necessary CSS and JavaScript references for DevExtreme controls.
Step 2: Define Your Form in the View
-
Create a form in your MVC view (
.cshtml
file) using DevExtreme’sdxForm
widget. -
This example defines a form with three fields: Name, Email, and Age. You can add more fields as needed.
Step 3: Prepare Your JSON String
-
Prepare your JSON string. For example:
-
You may retrieve this JSON data from a database, API, or static file, depending on your application.
Step 4: Write JavaScript to Populate the Form
-
Using JavaScript, parse the JSON string and set the form data dynamically.
-
This code parses the JSON string into a JavaScript object and uses DevExtreme’s
dxForm
method to populate the form data.
Step 5: Handle Data on the Backend
-
To handle the form data on the server, bind the form to a model in your MVC controller.
-
You can map the form fields to your
FormModel
and process the data accordingly.
Pro Tips and Best Practices
-
Always validate the JSON format: Before using JSON data, ensure that the data is properly formatted to avoid errors.
-
Use JavaScript async/await: If you’re fetching JSON from an API, ensure you use asynchronous methods for better performance.
-
Error handling: Implement error handling for scenarios where the JSON is invalid or missing key fields.
Variations and Customizations
-
Dynamic Data Population: If the form fields vary based on user selection or external conditions, you can update the JSON structure accordingly.
-
Additional Libraries: Consider using libraries like
axios
for API calls orjQuery
for simpler DOM manipulations. -
Customization for Multi-step Forms: If you have multi-step forms, use DevExtreme’s tab panels or wizard-like controls to enhance the user experience.
Serving Suggestions
-
Presentation: DevExtreme forms are designed to be responsive and clean by default. However, you can customize the design further using CSS to match your application’s theme.
-
Related Components: Pair your form with other DevExtreme widgets like
dxDataGrid
for displaying submitted data ordxButton
for form submission.
Nutritional Information
For those interested in the “nutritional” side of coding practices, this approach offers:
-
Simplicity: Easy integration between the frontend and backend.
-
Maintainability: Clear separation of concerns between form data and business logic.
-
Scalability: The technique can easily be extended to handle more complex forms.
Frequently Asked Questions (FAQs)
-
How do I populate the form with data from an API?
-
Use JavaScript’s
fetch()
or libraries likeaxios
to retrieve data and then populate the form as shown above.
-
-
What if my form fields change dynamically based on user selection?
-
You can dynamically generate JSON data on the backend and update the form using JavaScript when necessary.
-
-
How can I validate the data before populating the form?
-
Use JSON schema validation libraries or implement custom validation in your JavaScript code.
-
-
Can I use this method for complex forms with nested fields?
-
Yes! DevExtreme forms support complex structures, so you can map nested JSON objects to nested form fields as needed.
-
How to Populate DevExtreme MVC Form Data Using JSON String: A Developer’s Guide
Introduction
As web applications continue to evolve, so does the need to make forms more dynamic and responsive to user input. One of the most effective ways to achieve this is by populating form data with JSON strings. DevExtreme, a robust UI component library, offers a seamless way to bind JSON data to its MVC form controls, making this task more straightforward. In this guide, we will walk you through how to populate a DevExtreme MVC form with a JSON string, covering the core steps, challenges, and best practices.
DevExtreme’s form component is highly customizable and allows developers to work with different data types, including JSON, making it a versatile choice for web applications that need to present and edit data dynamically.
Ingredients
-
DevExtreme Form Control: A powerful form component for creating dynamic forms.
-
JSON Data String: The raw data format to be fed into the form.
-
JavaScript/jQuery: Essential tools for manipulating the DOM and interacting with the form.
-
MVC Backend (C#): For handling form submissions and interacting with the database.
Substitutions
-
For developers using different JavaScript frameworks (Angular, Vue.js, React), the principles remain similar, though the implementation may differ slightly based on the framework of choice.
Step-by-Step Instructions
Step 1: Set Up Your MVC Environment
-
Create a new MVC project in Visual Studio or your IDE of choice.
-
Install DevExtreme through NuGet or via CDN.
-
Include the DevExtreme CSS and JS files in your layout file.
Step 2: Define Your DevExtreme Form in the View In the view (.cshtml
file), define the structure of your form using the DevExtreme Form widget.
Step 3: Define and Parse Your JSON Data Create a JSON string that represents the data to be populated in the form.
Use JavaScript to parse the JSON string and dynamically populate the form.
Step 4: Handle Form Data on the Backend Create a model in your MVC controller to capture and process the form data.
Pro Tips and Techniques
-
Use Client-Side Validation: DevExtreme offers built-in validation rules that you can apply to form fields to ensure data integrity.
-
Error Handling: Add error handling mechanisms to deal with issues like incorrect JSON format or invalid data.
-
Form Customization: Customize form field types, labels, and layout based on your design requirements.
Variations and Customizations
-
Dynamic Fields: Populate the form with dynamic JSON data based on user actions or external inputs.
-
Nested JSON: Handle complex forms with nested JSON objects, allowing you to create multi-level forms that reflect hierarchical data structures.
Serving Suggestions
-
Showcase the Form: Integrate the form into your application with smooth transitions or animations for a better user experience.
-
Combine with Data Grids: After submitting the form, use a DevExtreme DataGrid to display submitted data in a table format.
Nutritional Information
-
Performance: DevExtreme’s form components are optimized for fast rendering and smooth user interactions.
-
Scalability: Whether you’re building a small form or a large, multi-step form, DevExtreme can scale with your project.
Frequently Asked Questions
-
Can I populate the form with data from a database? Yes, retrieve the data from your server and convert it into a JSON format to populate the form.
-
How do I validate JSON data? Use libraries like
json-schema
to validate the structure before using it to populate the form.
Populating DevExtreme MVC Form Data with JSON Strings: A Complete Guide
Introduction
Forms are integral to many web applications, especially when dealing with user input and data submission. One of the most efficient methods for populating form data is using JSON strings. This approach works especially well when combined with DevExtreme’s MVC form controls. In this guide, we will walk you through how to populate your DevExtreme MVC form using a JSON string, offering a simple yet powerful solution to handling dynamic data.
DevExtreme forms provide a robust UI control for handling various data types, and JSON integration makes it possible to load and manipulate complex data structures directly in the form. Whether you’re fetching data from an API or using static data, this method ensures smooth integration between your data source and UI controls.
Ingredients
-
DevExtreme MVC Form Control: A UI control designed for creating rich forms.
-
JSON String: The data you want to use to populate the form.
-
JavaScript/jQuery: Essential tools for handling DOM manipulations.
-
Backend MVC Controller (C#): To manage data on the server-side.
Substitutions
-
This method can also be adapted to work with other frameworks such as React, Vue, or Angular.Step-by-Step Instructions
Step 1: Set Up Your MVC Project
-
Create a new MVC project in Visual Studio and set up DevExtreme by installing it via NuGet or linking to the CDN.
-
Add the necessary DevExtreme resources in your layout view.
Step 2: Define the Form in Your View In your .cshtml
file, create a DevExtreme form control.
Step 3: Parse and Bind JSON Data Use a JSON string to populate the form data dynamically.
Step 4: Handle the Form Submission in the Controller In your MVC controller, create a method to handle form data submissions.
Pro Tips and Techniques
-
Async Data Loading: If your JSON data comes from an API, use async functions for smooth data loading.
-
Form Validation: Use DevExtreme’s validation methods to ensure the data entered is valid before submission.
Variations and Customizations
-
Multilingual Forms: Modify the JSON structure to support multiple languages and adjust form fields accordingly.
-
Complex Data: Use JSON arrays and nested objects for handling more complex data structures.
Serving Suggestions
-
Form Styling: Apply CSS or use DevExtreme themes to match the form with your web app’s design.
-
Form Interactivity: Add dynamic behaviors such as field highlighting or input validation to enhance user experience.
Nutritional Information
-
User-Friendly: DevExtreme’s easy-to-use forms simplify the process of creating dynamic, data-driven applications.
-
Compatibility: The method works seamlessly with modern browsers and frameworks.
Frequently Asked Questions
-
How do I retrieve JSON data from an external API? Use JavaScript’s
fetch()
orXMLHttpRequest
to get data from an API and populate the form. -
How can I handle nested data structures in JSON? DevExtreme forms support complex data models, including nested objects. Simply structure your JSON accordingly.
Closing Thoughts
Populating DevExtreme MVC forms with JSON is an essential technique for building data-driven web applications. By following this guide, you can easily implement dynamic forms that adapt to your data sources. Experiment with this powerful feature, and let it enhance your user interfaces today!
These two variations offer slightly different approaches and levels of detail, but both provide clear, actionable steps for integrating JSON data with DevExtreme MVC forms.