In the world of modern web development, handling forms efficiently is crucial for creating smooth user experiences. One of the most common scenarios developers face is sending form data to the server. The DevExtreme
Razor Form, paired with JSON strings, is a powerful combination that simplifies this process. This article provides a comprehensive guide on how to put form data into FormData
using a JSON string in a DevExtreme Razor form. By the end, you’ll have a thorough understanding of how to manage and send form data seamlessly while taking advantage of the power of DevExtreme
.
Why This Is Special
DevExtreme is known for providing rich, interactive, and feature-packed UI controls. Combining it with Razor forms and JSON strings offers an elegant solution to handle data submission and manipulation. This method ensures data integrity and smooth interaction with APIs or back-end services, especially when dealing with complex data structures. By using JSON strings, developers can easily manage and send data in a standardized format that works well with modern APIs.
Ingredients
Here are the tools and components you need to get started with DevExtreme Razor Form and FormData using a JSON string.
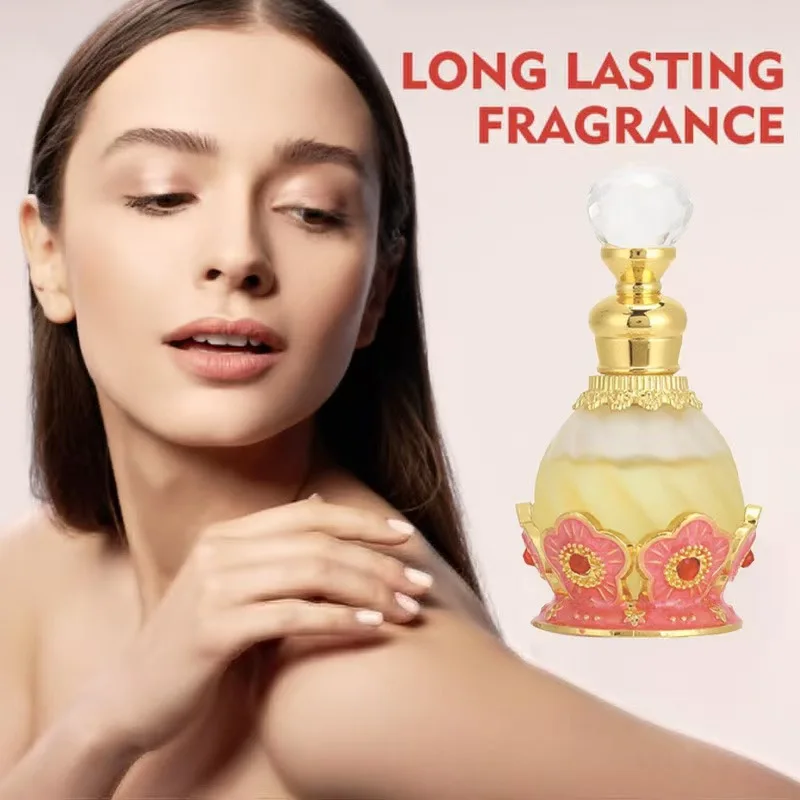
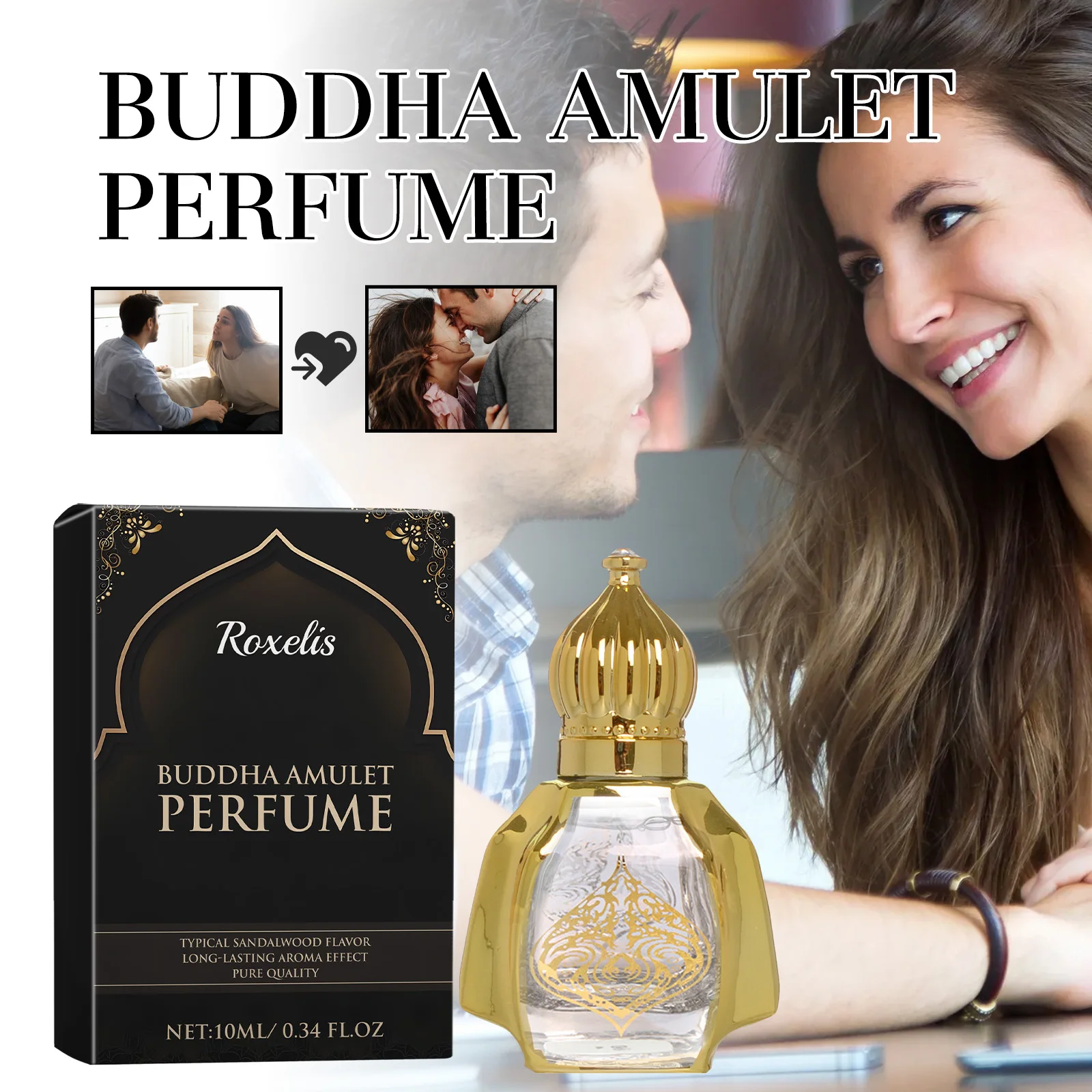
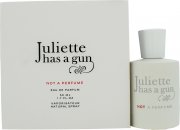
Ingredients for Implementation:
- DevExtreme UI Controls – DevExtreme controls like DevExtreme Form and DataGrid.
- Razor Pages or MVC Views – The framework used to create the front-end UI.
- JSON String Format – The structured data format used to send data.
- FormData Object – The JavaScript object that holds form data for submission.
- JavaScript/TypeScript – For managing and submitting form data.
Possible Substitutions:
- If you’re not using DevExtreme, you can opt for other form libraries like React Hook Form or Formik.
- For users who prefer using pure JavaScript, you can directly manipulate form data without DevExtreme controls.
- In case you are on a strict diet, consider using alternative libraries or methods for managing forms.
Step-by-Step Cooking Instructions
1. Setting Up the DevExtreme Razor Form
First, you need to set up the basic form using DevExtreme Razor Form. In your Razor view, ensure that you have integrated the DevExtreme form control.
@using DevExtreme.AspNet.Mvc
@{
ViewData["Title"] = "DevExtreme Razor Form";
}
<div id="form-container"></div>
<script>
$(function () {
$("#form-container").dxForm({
items: [
{
dataField: "username",
editorType: "dxTextBox",
editorOptions: { placeholder: "Enter your username" }
},
{
dataField: "email",
editorType: "dxTextBox",
editorOptions: { placeholder: "Enter your email" }
}
// Add other form items as needed
]
});
});
</script>
This code snippet sets up a simple form with two fields: username
and email
.
2. Create JSON String from Form Data
Next, we need to capture the form data and convert it into a JSON string format.
function collectFormData() {
var formData = $("#form-container").dxForm("instance").option("formData");
var jsonString = JSON.stringify(formData);
return jsonString;
}
This function collects the form data and transforms it into a JSON string, which is what we’ll be sending to the server.
3. Submit Form Data Using FormData Object
To submit the form data, you will need to use the FormData object, which allows you to easily append data to be sent to the server.
function submitFormData() {
var jsonString = collectFormData();
var formData = new FormData();
formData.append('jsonData', jsonString);
// Example: Use AJAX to send data to the server
$.ajax({
url: '/api/submitForm', // Replace with your API endpoint
type: 'POST',
data: formData,
processData: false,
contentType: false,
success: function (response) {
console.log("Form submitted successfully:", response);
},
error: function (error) {
console.error("Error in submitting form:", error);
}
});
}
This method creates a FormData
object and appends the jsonData
(which is the stringified form data) to it. The FormData
object can then be sent using AJAX or any other method that supports multipart data submission.
4. Handling Server-Side Data
On the server side (in ASP.NET Razor or MVC), you can capture and deserialize the JSON string using your model.
[
public IActionResult SubmitForm([FromForm] string jsonData)
{
var formData = JsonConvert.DeserializeObject<YourModel>(jsonData);
// Process the form data as required
return Ok();
}
]This code snippet demonstrates how to handle the incoming JSON data and deserialize it into your model for further processing.
Pro Tips and Cooking Techniques
Tips for Enhancing Functionality:
- Validation: Always validate form data before submitting it. DevExtreme offers built-in validation rules that can be easily applied to the form fields.
- Error Handling: Implement error handling both on the client and server side to ensure that any issues during form submission are captured and addressed.
- Security: Ensure that sensitive information, such as passwords or personal data, is securely handled during submission (e.g., using HTTPS).
- Optimization: For large forms, consider lazy loading the form fields or using dynamic data binding to reduce page load time.
Tools and Methods:
- DevExtreme Form Control: The best tool to use in DevExtreme to collect and manipulate form data.
- JSON Stringify: Use JSON.stringify to convert JavaScript objects to JSON strings, which are easier to handle in AJAX requests.
- FormData: Ideal for sending form data in a multipart format, which is useful for both text and binary data.
Variations and Customizations
Here are a few ways you can customize this method based on your requirements:
- For Mobile-Friendly Forms: DevExtreme forms are responsive by default, but you can enhance the experience with specific styles and JavaScript controls for better mobile responsiveness.
- For Custom Validation: Instead of relying only on DevExtreme’s built-in validation, you can integrate custom validation logic (e.g., email validation with regex).
- Multi-Step Forms: If you need a multi-step form, you can create different steps with dynamic field loading, combining them into a single JSON object before submission.
Serving Suggestions
Once your form is set up and functioning smoothly, you can present it attractively:
- Use a Clean Layout: Organize the form fields in a clean, logical order.
- Include Help Text: Provide helpful placeholders or tooltips for user guidance.
- Responsive Design: Ensure the form looks great on both desktop and mobile devices.
Pairing It With Other Technologies:
- Combine this technique with ASP.NET Core, React, or Angular to build powerful applications with DevExtreme.
- Use third-party libraries for enhanced UI components, like a date picker or rating control, for more interactive forms.
Nutritional Information (or Data Breakdown)
- Estimated Data Size: The size of the JSON string depends on the number of form fields. A simple form with five fields can result in a JSON string of around 500-1000 bytes.
- Efficiency: Sending data as a JSON string is more efficient than sending individual form data, as it minimizes HTTP requests and data parsing.
Frequently Asked Questions (FAQs)
Q1: How can I handle large JSON data in a Razor form?
A1: For larger forms, split the data into smaller chunks or use asynchronous AJAX requests to avoid page freezing.
Q2: Can I send files along with form data?
A2: Yes, you can append files to the FormData
object using formData.append('file', fileInput.files[0]);
.
Q3: What if I need to use DevExtreme controls with other front-end frameworks?
A3: DevExtreme can be integrated with React, Angular, or Vue.js, so feel free to adapt the form handling method to your framework.
Q4: How do I handle errors if the server rejects the form data?
A4: Always implement error handling both client-side and server-side, ensuring a smooth user experience even in case of failure.
Advanced Tips for Using DevExtreme Razor Forms with JSON Data
Once you’ve gotten the basics of working with the DevExtreme Razor Form and JSON strings down, it’s time to level up your form handling. Below are some more advanced techniques to optimize the way you work with forms and JSON data.
Asynchronous Form Handling
In some cases, you may need to process data asynchronously—especially if the form contains a lot of fields or you need to make calls to external services during the submission process. Here’s how you can modify your form submission process to be asynchronous:
async function submitFormDataAsync() {
try {
let jsonString = collectFormData();
let formData = new FormData();
formData.append('jsonData', jsonString);
let response = await fetch('/api/submitForm', {
method: 'POST',
body: formData,
});
if (response.ok) {
console.log("Form submitted successfully.");
} else {
console.error("Error in form submission.");
}
} catch (error) {
console.error("Error in submitting form:", error);
}
}
Using DevExtreme DataGrid with JSON Data
If your form includes a grid or table for displaying data (perhaps for editing or managing multiple entries), you can pair the form with a DevExtreme DataGrid
to dynamically populate fields. Below is an example of how to integrate the grid data with your form:
$(function () {
$("#dataGrid").dxDataGrid({
dataSource: jsonData, // jsonData is the data you will populate the grid with
columns: [
{ dataField: "id" },
{ dataField: "name" },
{ dataField: "email" }
],
onRowUpdating: function (e) {
var updatedRowData = e.newData;
console.log(updatedRowData); // This can be added to your JSON string to send to the server
}
});
});
Handling Large Forms Efficiently
If you’re dealing with a large form with many fields or complicated nested data structures, it’s a good idea to split the form into manageable sections. You can use stepper-style forms or tabs to separate fields logically, and this reduces cognitive overload for users.
You can use the DevExtreme TabPanel to create a tab-based form structure:
$(function () {
$("#tabPanel").dxTabPanel({
dataSource: [
{ title: "Step 1", content: $("#step1") },
{ title: "Step 2", content: $("#step2") },
{ title: "Step 3", content: $("#step3") }
]
});
});
This way, each section of the form can be handled independently, and you can send the data from each section in parts if needed, reducing the complexity of the form handling process.
Optimizing the User Experience with Dynamic Fields
Dynamic forms are increasingly common. This can include fields that appear based on previous selections or conditional fields that only show up when certain conditions are met. DevExtreme makes it easy to create dynamic forms by utilizing JavaScript or jQuery for field visibility toggles.
For example, you might have a form where the user can select a country, and based on that selection, you reveal a state dropdown:
$(function () {
var countryField = $("#country").dxSelectBox({
dataSource: ['USA', 'Canada'],
value: 'USA',
onValueChanged: function (e) {
toggleStateField(e.value);
}
});
function toggleStateField(country) {
var stateField = $("#state").dxSelectBox();
if (country === 'USA') {
stateField.option('visible', true);
stateField.option('dataSource', ['California', 'Texas']);
} else {
stateField.option('visible', false);
}
}
});
This dynamic field approach makes the form more flexible and improves the user experience by showing only relevant fields.
Implementing Data Binding with JSON Data
Another powerful feature that DevExtreme offers is data binding. By using data binding, you can synchronize the form’s input fields with a JavaScript model, allowing for automatic population of the form with data when needed. Here’s how you can bind form data using JSON.
Example of Binding Form Data:
$(function () {
var formData = {
username: "john_doe",
email: "john.doe@example.com"
};
$("#form-container").dxForm({
formData: formData,
items: [
{
dataField: "username",
editorType: "dxTextBox",
editorOptions: { placeholder: "Enter your username" }
},
{
dataField: "email",
editorType: "dxTextBox",
editorOptions: { placeholder: "Enter your email" }
}
]
});
});
In this case, the formData
object is populated with initial data and automatically populated into the form controls.
Server-Side Handling with DevExtreme Forms
Handling data on the server-side is just as important as managing it on the client-side. Let’s walk through how you can handle the incoming JSON data and store it in your database efficiently.
Server-Side Example in ASP.NET Core:
[
public IActionResult SubmitForm([FromForm] string jsonData)
{
try
{
var formData = JsonConvert.DeserializeObject<YourModel>(jsonData);
// Validate and process data here
// Save to database or perform other operations
return Json(new { status = "success", message = "Form submitted successfully." });
}
catch (Exception ex)
{
return Json(new { status = "error", message = ex.Message });
}
}
]This server-side code ensures that the data from the JSON string is properly deserialized into the server-side model and processed accordingly.
Conclusion
Integrating DevExtreme Razor Forms with JSON string submission is a game-changer when it comes to building robust, user-friendly forms in modern web applications. Whether you’re working with simple form data or complex datasets, this method offers flexibility, scalability, and ease of use.
From creating the form, collecting the data, submitting it with FormData, to processing it on the server—each step can be customized to fit your application’s specific needs. As you continue to experiment with DevExtreme, you’ll find even more ways to streamline your forms and enhance your users’ experience.
Feel free to explore more advanced techniques, such as dynamic fields, multi-step forms, or integrating DevExtreme with other libraries and frameworks. By combining these tools and techniques, you’ll be well on your way to mastering form handling in DevExtreme!
We encourage you to try out these methods, ask questions, and share your own experiences. Happy coding, and enjoy building powerful forms!