Fading a LED on the ESP32-S3 is an exciting way to explore PWM (Pulse Width Modulation) and dive deeper into the world of embedded systems programming. The ESP32-S3, a powerful microcontroller from Espressif, offers a great platform for experimenting with LED control. Whether you’re a beginner looking to learn or an experienced maker aiming to refine your skills, this guide will walk you through the steps necessary to achieve a smooth fading effect on a LED.
The fading effect involves gradually changing the brightness of the LED, creating a smooth transition from dim to bright and vice versa. This effect is popular in various projects, including ambient lighting, visual indicators, and decorative elements. By the end of this guide, you’ll have the skills to program the ESP32-S3 to control LEDs with a fade effect.
Ingredients
To get started with fading a LED on the ESP32-S3, you’ll need the following ingredients:
- ESP32-S3 Development Board (any model will work, but ensure it has sufficient GPIO pins)
- LED (any standard 5mm LED, preferably a white or RGB LED for better visibility)
- 220Ω Resistor (to protect the LED from excess current)
- Breadboard and Jumper Wires (for easy prototyping and connections)
- Arduino IDE (installed and set up for ESP32-S3 development)
Substitutions/Notes:
- If you prefer, you can use a different microcontroller (such as ESP32 or ESP8266), but the code might need slight adjustments.
- If you’re working on a project with multiple LEDs, ensure that your power supply and resistors can handle the total current.
Step-by-Step Cooking Instructions
Follow these steps to create a smooth LED fading effect on the ESP32-S3.
-
Prepare the Circuit:
- Connect the long leg (anode) of the LED to any available GPIO pin (let’s use GPIO 13 for this example).
- Connect the short leg (cathode) of the LED to one side of the 220Ω resistor.
- Connect the other side of the resistor to the GND pin on the ESP32-S3.
-
Setup Arduino IDE for ESP32-S3:
- Make sure you have the Arduino IDE installed on your computer. If not, download it from
- Install the ESP32 board support by going to File > Preferences and adding this URL to the “Additional Boards Manager URLs” field:
https://dl.espressif.com/dl/package_esp32_index.json
. - Then, go to Tools > Board > Board Manager, search for “ESP32,” and install the latest version.
-
Write the Code: Open a new sketch in Arduino IDE and write the following code:
-
Upload the Code to ESP32-S3:
- Select the correct board and port from Tools > Board and Tools > Port.
- Click the Upload button to compile and upload the code to your ESP32-S3.
-
Observe the Fading Effect:
- Once the code is uploaded successfully, you should see the LED gradually fade in and out in brightness. This will repeat continuously.
Tips:
- Ensure your LED is correctly connected to prevent it from burning out due to excessive current.
- The
delay()
function controls the speed of the fade; increasing the delay will slow the fading process.
Pro Tips and Cooking Techniques
-
PWM Frequency: You can adjust the PWM frequency using the
ledcWriteTone()
function, which might offer smoother fading at higher frequencies. -
Smoothing the Fade: If the LED fades too abruptly, try increasing the delay time or using a non-linear curve for brightness adjustment.
-
Using RGB LEDs: If you use an RGB LED, you can adjust the color by fading each color channel (Red, Green, Blue) separately.
Example:
This would allow you to create gradient color fades instead of just white.
Variations and Customizations
-
RGB Fade Effect: If you are using an RGB LED, you can fade each color channel (red, green, blue) to create a colorful fade effect. You can create smooth transitions between different colors by fading each channel individually.
-
Using Multiple LEDs: To create a more complex fade pattern, you can connect multiple LEDs to different pins and fade them in and out in a sequence. This can create a chase or strobe effect.
-
Different Speeds and Intervals: Adjust the delay or use a timer interrupt to control the fade speed programmatically, offering a dynamic and customizable fade.
Serving Suggestions
Once your ESP32-S3 is successfully controlling the LED, you can display it in various projects, such as:
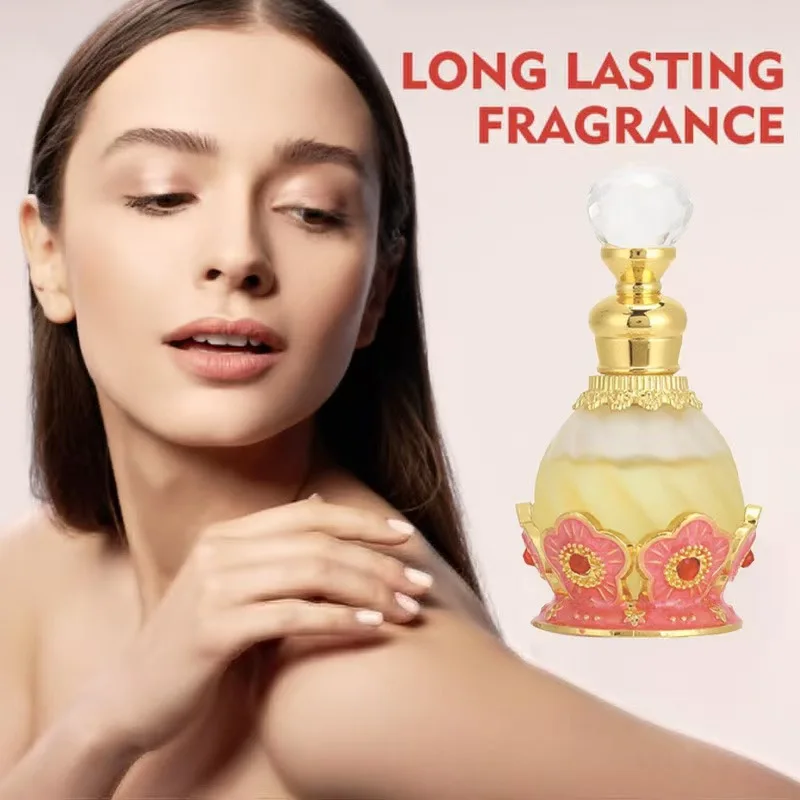
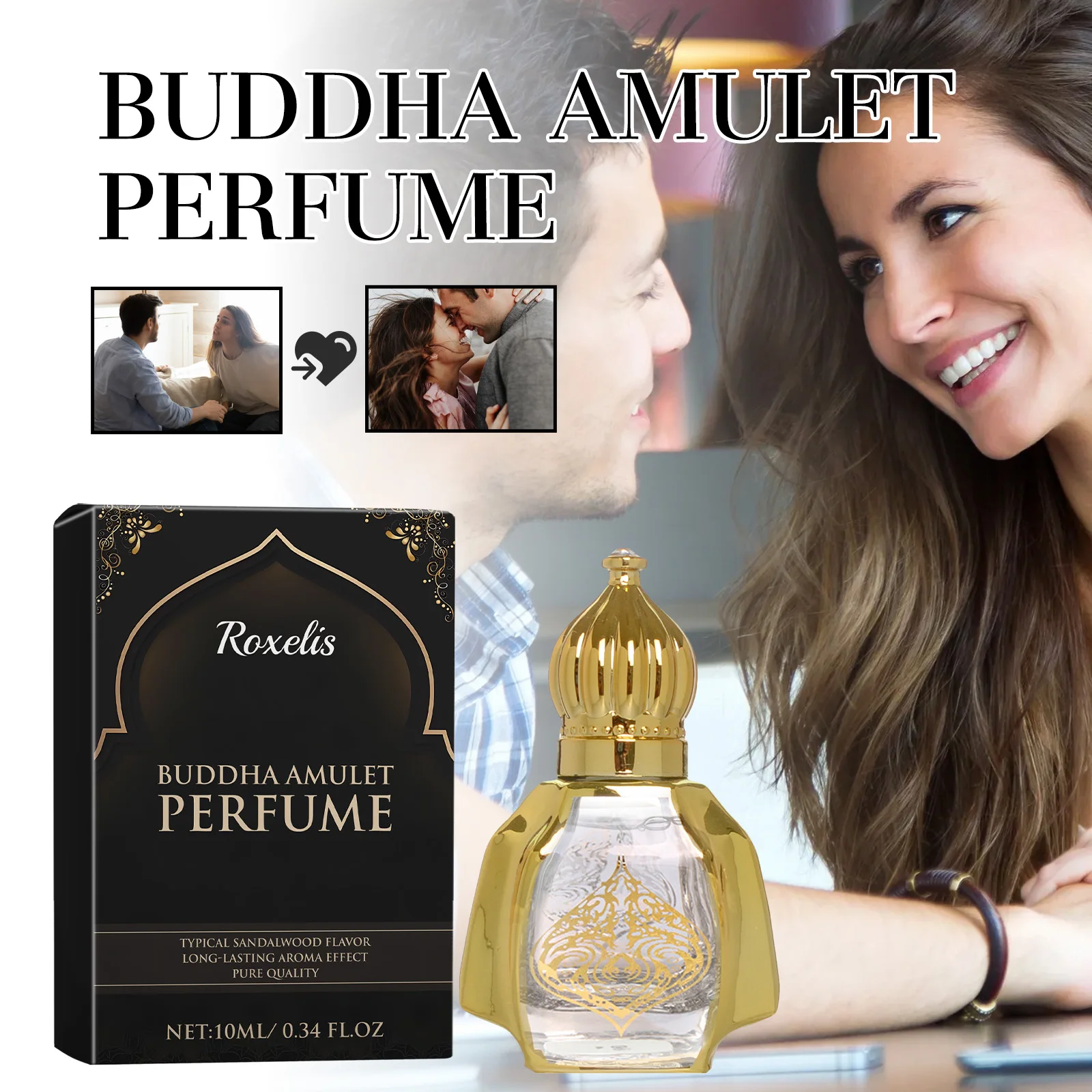
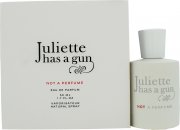
- Ambient Lighting: Use the LED fade effect to create soft lighting for smart home projects or decorative purposes.
- Indicators: Use fading as a visual indicator for system statuses, like a battery low alert or network connection status.
- Art Installations: Incorporate fading LEDs into interactive art installations, where the lights change in response to user input or environmental factors.
Nutritional Information (Technical Specifications)
While the fading LED effect doesn’t involve food, here’s a technical breakdown of the power consumption for your ESP32-S3 setup:
- LED Consumption: Each 5mm LED typically consumes around 20mA of current at full brightness.
- ESP32-S3 Consumption: The ESP32-S3 typically draws 160mA during normal operation, but this varies depending on the number of peripherals in use.
Frequently Asked Questions (FAQs)
1. Why is my LED not fading smoothly?
- Ensure that you are using a proper PWM signal and not simply turning the LED on or off. Adjust the delay time to smooth out the transitions.
2. Can I use a different GPIO pin?
- Yes, you can use any available GPIO pin on the ESP32-S3 that supports PWM. Just update the pin number in the code.
3. How can I make the fade faster or slower?
- Adjust the
delay()
value in the loop. A lower value will make the fade faster, while a higher value will slow it down.
4. What happens if I use a higher voltage LED?
- Always use the appropriate resistor to limit current to avoid damaging the LED.
5. Can I run multiple LEDs with the same fade effect?
- Yes, by controlling multiple pins with similar PWM outputs, you can achieve synchronized fading with several LEDs.
How to Get a LED to Fade on ESP32-S3: Advanced Guide for Makers
Introduction
Controlling LEDs with smooth fading effects opens up numerous possibilities for creative projects. Whether you’re designing ambient lighting, a notification system, or simply enhancing your skills with microcontrollers, learning how to fade a LED using the ESP32-S3 will give you both foundational knowledge and creative freedom. The ESP32-S3 is a powerhouse for controlling LEDs with precision, thanks to its advanced PWM capabilities.
In this advanced guide, we’ll walk you through the steps of not just fading a LED but optimizing it for performance, creating smooth transitions, and experimenting with different patterns. Get ready to dive deeper into the world of embedded systems!
Ingredients
You will need the following tools:
- ESP32-S3 Development Board (highly recommended for advanced projects)
- LED (standard or RGB)
- 220Ω Resistor (to protect your LED)
- Breadboard and Jumper Wires
- Arduino IDE (set up for ESP32-S3)
- Optional: Transistor or MOSFET (if you want to control multiple LEDs or higher-powered LEDs)
Step-by-Step Cooking Instructions
-
Basic Circuit Setup:
- Place the LED onto the breadboard. Connect the anode (longer leg) to GPIO 13 and the cathode (shorter leg) through the 220Ω resistor to the GND pin of the ESP32-S3.
-
Set up Arduino IDE:
- In the Arduino IDE, make sure to have the ESP32-S3 board selected and the right port chosen. If you’re not sure about how to do this, consult Espressif’s tutorials to ensure you have your setup configured correctly.
-
Write the Advanced Code: For smoother transitions, we’ll use ESP32-S3’s
ledcWrite()
function for PWM control instead ofanalogWrite()
. Here’s the code: -
Upload the Code:
- Upload the code to your ESP32-S3 via Arduino IDE. The LED will fade smoothly from off to full brightness and back.
Pro Tips and Cooking Techniques
- High-Resolution PWM: Using 8-bit resolution (0-255) allows for smooth transitions. If you want more granular control, consider using 10-bit (0-1023).
- Non-linear Fade Effects: Instead of linearly fading from 0 to 255, you can use mathematical functions like sine or logarithmic curves to create more natural fades, especially for slow, calming lighting effects.
Variations and Customizations
- RGB LED with Smooth Color Transitions: Use three PWM channels to control each color channel of an RGB LED and program them to transition smoothly between colors.
- Multiple LEDs: With the ESP32-S3’s multiple PWM channels, you can control several LEDs simultaneously or in a sequence, allowing you to create more complex lighting effects like a “heartbeat” pattern or a dynamic wave effect.
Serving Suggestions
- Smart Lighting Systems: Use multiple LEDs to create lighting effects that change based on time of day or events.
- Art Installations: Add LEDs to interactive art installations to make them react to user input or environmental changes.
Nutritional Information
- LED Power Consumption: Typical 5mm LEDs consume around 20mA when at full brightness.
- ESP32-S3 Consumption: The ESP32-S3 typically draws around 160mA but can vary depending on peripherals and tasks.
Frequently Asked Questions
Q: Can I use different GPIO pins for multiple LEDs?
- Yes! You can use multiple GPIO pins and control each with its own PWM channel.
Q: How can I make the LED fade more slowly or quickly?
- Adjust the delay in the loop, or better yet, modify the PWM frequency for finer control.
Closing Thoughts
Mastering PWM control on the ESP32-S3 to fade a LED opens doors to endless creative possibilities. From making smooth lighting transitions to building complex visual effects, the skills you gain from this project are transferable to many advanced applications. Experiment with different techniques, and don’t hesitate to push the boundaries of what you can create!