Greenfoot is a great platform for learning programming through interactive visuals. If you’re working on a project and need to find the index value of an object, it’s important to understand how Greenfoot handles objects in its world. Knowing the index value allows you to manipulate objects with precision and control. Here’s a simple guide to help you get started.
What is an Index Value in Greenfoot?
The index value of an object refers to its position within the list or array of objects in Greenfoot’s world. In programming, especially in object-oriented environments like Greenfoot, objects are stored in data structures such as lists or arrays. The index helps you identify where an object is located within these structures.
Greenfoot uses an object-oriented approach, meaning objects are the primary entities within the world. To interact with or manipulate these objects, it’s crucial to understand their position or index value. You can use this index value to access and modify the object’s properties, behavior, or location.
Why is Identifying the Index Value Important?
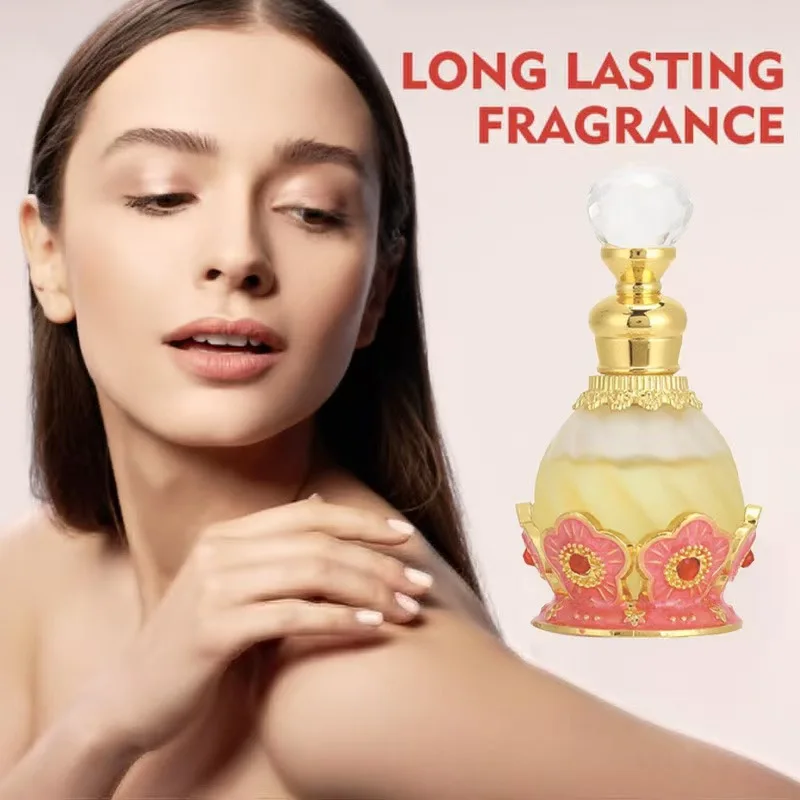
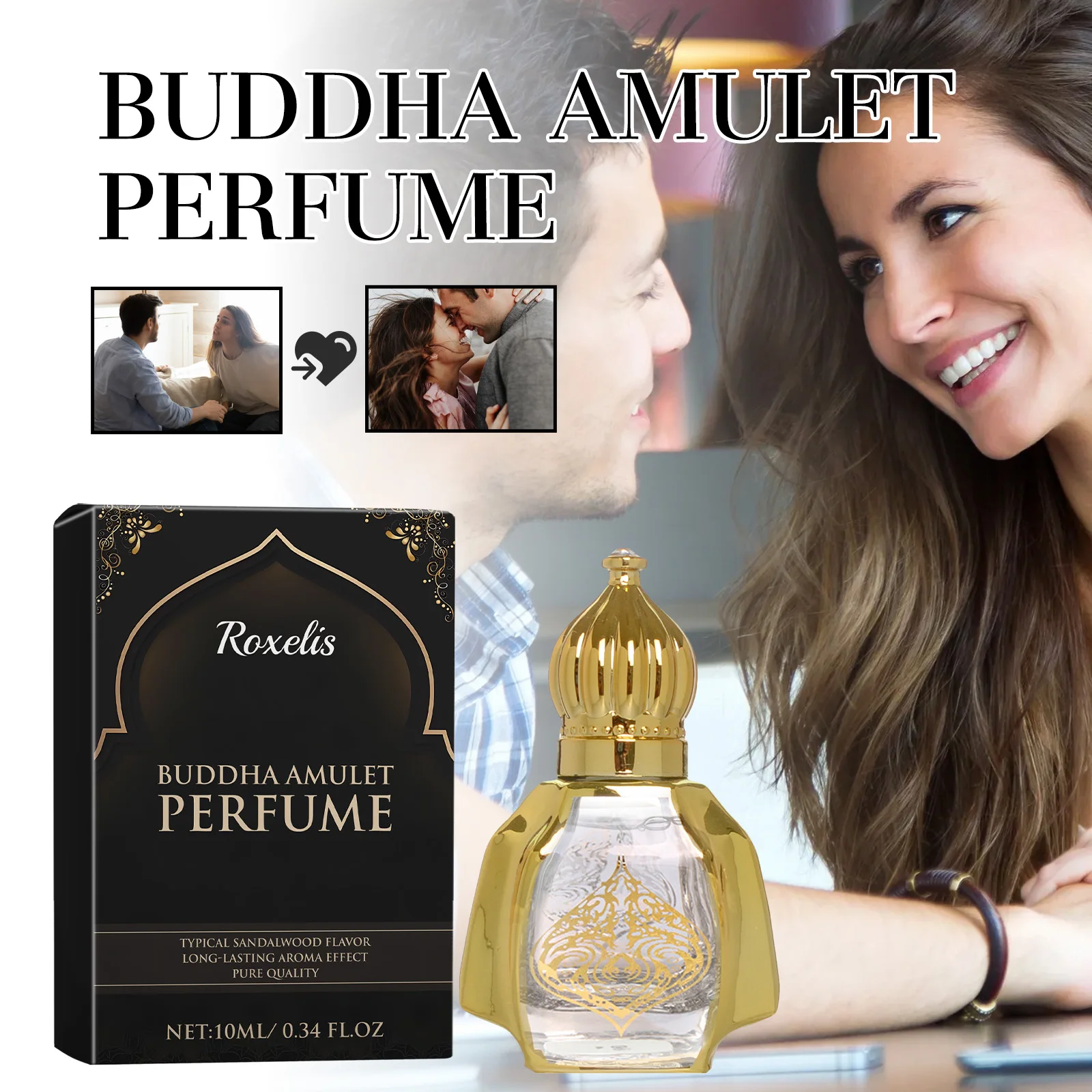
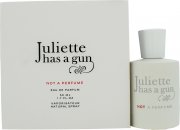
Understanding the index value of an object in Greenfoot allows you to:
- Access objects efficiently within the world.
- Create dynamic interactions between objects based on their position.
- Implement complex logic that depends on the position of an object.
When you know the index value, it becomes easier to handle multiple objects in Greenfoot, especially when you are working with large numbers of them.
How Greenfoot Handles Objects in the World
In Greenfoot, the world is populated by objects that you create using classes. These objects are usually instances of a specific class, and they live within the world until they are removed. These objects can interact with one another based on their properties, methods, and positions.
The objects are stored in a list or array-like structure, and their index values are assigned automatically by the system when the objects are added to the world. These index values represent the position of each object in the list and help the system manage objects effectively.
Steps to Identify the Index Value of an Object in Greenfoot
Identifying the index value of an object in Greenfoot is relatively simple, but you need to know the right methods and concepts to get the correct result. Below are some practical ways to identify the index value of an object:
- Use the
getObjects()
Method: This method is useful for retrieving a list of objects of a specific class. The objects are returned as an array, and you can use their position in the array as the index. For example, if you have a class calledPlayer
, you can get all instances of this class usinggetObjects(Player.class)
. - Iterate through the Object List: Once you have an array of objects, you can loop through them using a
for
loop or an enhancedfor
loop to check the index. This is particularly useful when you want to find the exact position of a specific object. - Use
getObjectAt(x, y)
Method: If you want to find the object at a specific location in the world, thegetObjectAt(x, y)
method can be used. This method helps you identify objects based on their coordinates, which is essential when you want to pinpoint an object’s index based on its location. - Work with Arrays and Lists: If you are using arrays or lists explicitly to manage objects in your world, the index of the object can be found using standard array or list methods. You can use
indexOf()
in a list or simply use the array index when working with arrays.
Practical Examples of Finding Index Values
Here are a couple of examples that show how you can find the index of an object in Greenfoot:
-
Example 1: Finding Index with getObjects()
-
Example 2: Finding Object at a Specific Position
In these examples, you can see that identifying an object’s index is done using built-in Greenfoot methods, such as getObjects()
and getObjectAt(x, y)
.
Best Practices for Managing Objects in Greenfoot
When working with objects in Greenfoot, it’s essential to follow best practices to keep your code clean and efficient:
- Organize Your Objects: Keep track of your objects in arrays or lists. This allows you to easily manage them and access their index values whenever needed.
- Use Loops for Efficient Access: If you have multiple objects, iterate through them using loops to process each object’s index efficiently.
- Avoid Hardcoding Indexes: Instead of manually specifying index values, it’s better to use dynamic methods to find the index of an object based on its properties or location. This makes your code more flexible and adaptable to changes.
- Consider Object Types and Classes: If you have multiple types of objects, it’s important to manage them by their classes or types. Greenfoot allows you to filter objects based on their class, which helps to avoid confusion.
Troubleshooting Index Issues
Sometimes, you might encounter problems when trying to identify the index value of an object. Here are a few common issues and how to resolve them:
- Null Objects: If you are trying to access an object at a certain index but the object doesn’t exist, you might get a
null
value. Make sure that the object you are trying to access is not null before processing. - Out-of-Bounds Errors: When accessing arrays or lists, make sure that the index you are trying to access is within the bounds of the array or list. If you try to access an index that is out of range, it will result in an error.
- Objects Not Found: If you cannot find an object at a particular index, check that the object exists in the list or array and that it was properly added to the world.
Understanding the Role of Index Values in Greenfoot Projects
Index values are crucial when it comes to managing objects within your Greenfoot world. Whether you are developing a simple game or a more complex simulation, understanding how to identify and work with these index values will significantly improve the efficiency of your programming.
In Greenfoot, objects do not just appear randomly; they are managed systematically, often in an array or list, where each object is assigned an index value. This helps the system organize objects so you can easily interact with or manipulate them. For example, if you are building a game with multiple characters, knowing the index value allows you to locate each character in the array and apply logic like movement or collision detection.
How Index Values Enhance Object Interactions in Greenfoot
When you work with objects in Greenfoot, they aren’t just static entities—they interact with one another in the world. Index values allow you to direct how these objects interact, such as:
-
Movement and Positioning: You can use the index to find out where each object is located. For example, if you are controlling a player character, you can update its position based on the object’s index value.
-
Collision Detection: If you need to detect when two objects collide, knowing their index values can help you track their movement and interaction. By comparing the index of objects, you can determine when two objects meet.
-
Game Logic and Object Behavior: When managing multiple objects, you may want to change their behaviors depending on their position or relationship to other objects. Knowing their index helps you isolate objects for specific interactions, such as checking whether a particular enemy is in range or if an item is collected.
Using the Index to Filter and Organize Objects
When you have many objects in the world, it can get tricky to manage them all efficiently. Index values help by allowing you to filter and organize objects based on their class or properties.
-
Object Filtering: In some cases, you may only want to interact with certain objects in your world, such as all the enemies or all the obstacles. By identifying their index, you can filter objects of a specific type and perform actions on them.
-
Object Management: If you’re working with a large number of objects, like multiple instances of a class, you can use their index values to manage them in batches. For instance, you could remove all obstacles from the world by referencing their indices, which is much more efficient than checking each object individually.
Practical Example: Tracking and Updating Multiple Objects
Suppose you have a Greenfoot world filled with multiple moving objects. Here’s how you could use the index values to track and update these objects.
This example demonstrates how knowing the index of each enemy in the list allows you to move each enemy object and keep track of its position.
Managing Dynamic Object Lists
In dynamic Greenfoot projects, where objects may be added or removed during gameplay, the index value plays a critical role in managing changes. For example, if an object is removed from the world, its index may shift, affecting the position of other objects in the array or list. Here’s how you can manage such changes:
-
Removing Objects: If you remove an object from the world, the objects that follow it in the array will shift down by one index. It’s important to account for these shifts when processing objects to avoid errors.
-
Adding New Objects: When adding new objects to the world, the system will automatically assign them an index based on their position in the list. However, you may need to reorder objects if their index is used in certain calculations or interactions.
Best Practices for Working with Index Values in Greenfoot
To ensure you’re using index values effectively in Greenfoot, consider the following best practices:
-
Use Arrays or Lists for Object Management: Arrays and lists are excellent tools for managing objects in Greenfoot. They allow you to store objects in a way that is easy to access and manipulate based on their index.
-
Efficiently Handle Large Numbers of Objects: When working with a large number of objects, always keep track of their indices to improve performance. Iterating through objects using their index values will help optimize your code.
-
Avoid Index Overlaps: Ensure that you avoid conflicting index values when objects are added or removed from lists. This will prevent errors such as attempting to access an object at an invalid index.
Conclusion
Identifying the index value of an object in Greenfoot is a valuable skill that helps you manage and interact with objects effectively. By using methods like getObjects()
and getObjectAt(x, y)
, you can find the position of objects in your world and utilize this information to create dynamic interactions. Remember to follow best practices when organizing your objects and avoid common issues like null references or out-of-bounds errors. With these tips, you’ll be able to manage objects with precision and enhance your Greenfoot projects.